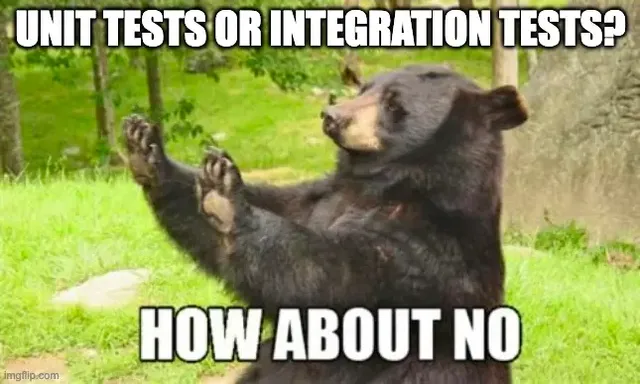
As software development becomes more complex, the need for testing grows. Testing is an integral part of the software development lifecycle, and it is essential for ensuring the reliability, functionality, and quality of the software being developed. There are several types of testing that software developers use, but two of the most common are unit testing and integration testing.
What is Unit Testing?
Unit testing is the process of testing individual units of code in isolation. A unit is the smallest testable part of an application. It could be a method, a function, or a class. The goal of unit testing is to ensure that each unit of code performs as expected and meets the requirements defined in the specifications. Unit testing is typically done by developers during the development phase of the software development lifecycle.
Let's consider an example of unit testing in Java. Suppose we have a class called Calculator
that performs basic arithmetic operations. We want to test the add
method of the Calculator
class. Here's what the code for the test might look like:
1@Test
2public void testAdd() {
3 Calculator calculator = new Calculator();
4 int result = calculator.add(2, 3);
5 assertEquals(5, result);
6}
In this example, we're testing the add
method of the Calculator
class by creating an instance of the Calculator
class and calling the add
method with the arguments 2
and 3
. We then use the assertEquals
method to check that the result of the add
method is 5
, which is what we expect.
What is Integration Testing?
Integration testing is the process of testing how different units of code work together. It is designed to identify problems that arise when units of code interact with each other. The goal of integration testing is to ensure that the different units of code can communicate and work together as expected. Integration testing is typically done after unit testing and before system testing.
Let's continue with our example from before. Suppose we have a class called RemoteCalculatorService
that is hosted on an application server and uses the Calculator
class to perform operations. We want to test the add
method of the RemoteCalculatorService
class. Here's what the code for the test might look like:
1@Test
2public void testAdd() {
3 RemoteCalculatorService calculatorService = new RemoteCalculatorService("127.0.0.1");
4 int result = calculatorService.add(2, 3);
5 assertEquals(5, result);
6}
In this example, we're testing the add
method of the RemoteCalculatorService
class by creating an instance of the RemoteCalculatorService
class and calling the add
method with the arguments 2
and 3
. The add
method of the RemoteCalculatorService
class then calls the add
method of the Calculator
class to perform the actual calculation. We're testing that the add
method of the RemoteCalculatorService
class works correctly with the add
method of the Calculator
class.
Difference between Unit-testing and Integration Testing
The main difference between unit testing and integration testing is that unit testing tests individual units of code in isolation, while integration testing tests how different units of code work together. Unit testing is focused on the functionality of each unit of code, while integration testing is focused on the interactions between different units of code.
Proposal to Rename Testing Methods
The names "unit testing" and "integration testing" have caused confusion for some time. Some developers have mistaken "unit testing" to mean "testing with small data sets" or "testing with mock objects," while others have thought "integration testing" meant "testing with large data sets" or "testing with real objects."
To eliminate confusion, we propose renaming these testing methods. Instead of "unit testing" and "integration testing," we suggest using the names "I/O Free testing" and "I/O included testing," respectively.
"I/O Free testing" refers to testing code in isolation from external dependencies such as databases, file systems, and network connections. The focus of I/O Free testing is on the logic and functionality of the code itself, without any external interference.
"I/O included testing," on the other hand, involves testing the interactions between different units of code and their external dependencies. The focus of I/O included testing is on how the code interacts with its environment and external resources.
By using these names, we can eliminate the confusion that has arisen from the current names and make it easier for developers to understand the purpose and scope of each type of testing. It's important to note that these new names are not intended to replace the current terms in all cases but rather to provide a clearer way to distinguish between the two types of testing.
Let’s talk!
We’re confident we can supercharge your software operation. Our unique products and services will delight you.
Read more:
Unit tests or Integration Tests?
As software development becomes more complex, the need for testing grows. Testing is an integral part of the software de...
AI is the missing piece of the Productivity Puzzle
Today, I’d like to plea that Artificial Intelligence (AI) is the missing piece of the productivity puzzle, revolutionizi...
Say Goodbye to Frustration: ZEN Software's Plugin Makes Picture Labeling a Breeze!
WordPress empowers businesses, creative enthusiasts, and content creators with its user-friendly interface and extensive...
Programmer frustration: Yak Shaving
The term "yak shaving" in programming originated from an episode of the popular 1990s cartoon "Ren & Stimpy." The episod...
Amazon Prime Video swaps Microservices for Monolith: 90% Cost Reduction
Recently, Amazon Prime Video published a surprising article [revealing how they saved 90%](https://www.primevideotech.co...
Remote Development in the Cloud
Remote development has become increasingly popular in recent years. It allows developers to take advantage of powerful c...